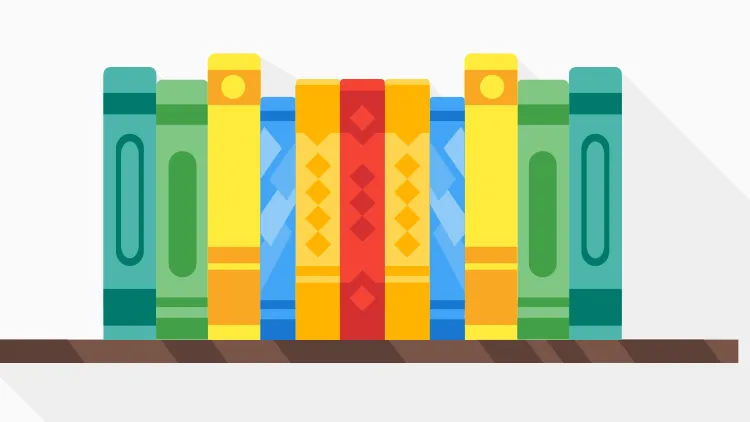
Harnessing the Power of Jenkins Shared Libraries
Table of Contents
- What is a Jenkins Shared Library?
- Configuring Jenkins
- Creating and Using Custom Steps
- The Power of Shared Libraries
Jenkins Shared Libraries are an incredible resource for anyone working with CI/CD pipelines, allowing for the reusability of code and a clean, efficient structure in your Jenkinsfiles. In this blog post, we’ll break down the steps for how to use Jenkins Shared Libraries, with the goal of streamlining your Jenkins workflow.
What is a Jenkins Shared Library?
Jenkins Shared Libraries are a collection of independent groovy scripts that can be used by any job in the Jenkins instance. They act as a means to reuse scripts across multiple Jenkinsfiles, making it easy to maintain and manage the code.
Creating a Shared Library
- Initialize a Git Repository: Shared libraries are pulled into Jenkins through source control. Hence, the first step is to initialize a Git repository and push it to a central repository (like GitHub).
- Create the Directory Structure: Jenkins shared libraries need a specific directory structure. A typical structure looks like this:
(root)
+- src # Groovy source files
| +- org
| +- foo
| +- Bar.groovy # for org.foo.Bar class
+- vars
| +- foo.groovy # for global 'foo' variable
| +- foo.txt # help for 'foo' variable
+- resources # Resource files (non-Groovy)
+- org
+- foo
+- bar.json # static helper data for org.foo.Bar
src
is for your Groovy source files, vars is for your global scripts, and resources is for your static resources.
- Develop Your Scripts: You can now develop your groovy scripts under the src or vars directory.
Here is an example for a groovy script in the vars directory, deploy.groovy:
def call(body) {
// evaluate the body block, and collect configuration into the object
def config = [:]
body.resolveStrategy = Closure.DELEGATE_FIRST
body.delegate = config
body()
// now use the configuration map
if (config.environment == 'production') {
echo "Deploying to production"
} else {
echo "Not production deployment"
}
}
Configuring Jenkins
- Add Shared Library to Jenkins: Go to Jenkins home > Manage Jenkins > Configure System > Global Pipeline Libraries, then fill in your details under the Library section.
- Name: Any name of your choice
- Default version: Specify a version (branch name, tag, commit sha)
- Retrieval method: Select Modern SCM
- Source Code Management: Choose Git, then enter your Repository URL
- Use Shared Library in Jenkinsfile: You can now load your shared library into your Jenkinsfile. There are two ways to do this:
- Implicit: By going into the Jenkins configuration, and under Global Pipeline Libraries, checking the “Load implicitly” option, the library will be loaded automatically in every Jenkinsfile without explicitly defining it.
@Library('your-library-name') _
* Explicit: In the Jenkinsfile itself, you can load the shared library:
@Library('your-library-name') import org.foo.Bar
You can also load a specific version of the library:
@Library('your-library-name@version') import org.foo.Bar
- Using the Shared Library: Now that your shared library is loaded, you can call the methods in your Jenkinsfile. For the deploy method we wrote earlier, it can be called like so:
deploy {
environment = 'production'
}
The above script will call the deploy function we’ve defined in the Shared Library, passing the ‘production’ environment as a parameter.
Creating and Using Custom Steps
One of the most common uses of Jenkins Shared Libraries is the creation of custom steps. Let’s walk through creating a custom step.
- Create the Custom Step: You’d need to create a file in the vars directory in your Shared Library repository. Let’s create a file named customStep.groovy. This file should contain a call function, which is what will be run when the step is called. Here’s an example:
def call(String name) {
// This is the code that will be run when customStep is called.
echo "Hello, ${name}"
}
- Use the Custom Step: Now you can use the customStep in your Jenkinsfile. If you’ve set your Shared Library to load implicitly, you can simply call it as if it were a step:
pipeline {
agent any
stages {
stage('Hello') {
steps {
customStep('World')
}
}
}
}
This will print “Hello, World” in the console output.
The Power of Shared Libraries
As you can see, Jenkins Shared Libraries provide a powerful way to share code between Jenkins pipelines, allowing for code reuse, reduced duplication, and easier maintenance. It’s a feature that, when properly utilized, can greatly improve your CI/CD pipelines.
Remember that each function in your Shared Libraries should be as small and specific as possible. This will make them easier to use and reuse across different pipelines. Also, as they are code, don’t forget to write tests to ensure they work as expected.
In conclusion, Shared Libraries are an essential part of Jenkins that everyone using Jenkins should be familiar with. They might seem a bit complicated at first, but once you get the hang of it, they can make working with Jenkins a lot smoother and more enjoyable.