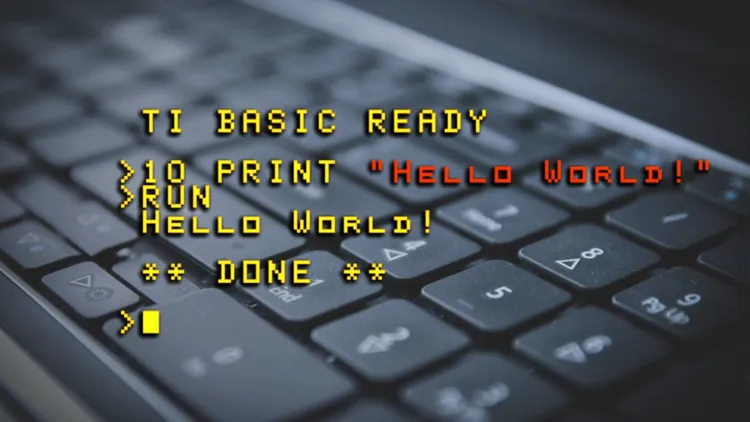
Colorize Your Shell Scripts: Printing Colored Output in Shell
Table of contents
- Introduction:
- Understanding ANSI Escape Codes
- Creating Colored Output in Shell Scripts
- Reusable Functions for Colored Output
- Conclusion:
Introduction:
Breathe life into your shell scripts by adding colors to your output. By using ANSI escape codes, you can customize the appearance of text displayed in the terminal. In this blog post, we’ll cover how to create colorful outputs in shell scripts, as well as how to create reusable functions for various text styles.
Understanding ANSI Escape Codes
ANSI escape codes are sequences of characters used to control the appearance of text in the terminal. They are often used to change the color, style, and background of the text. The basic syntax for an ANSI escape code is:
\033[{attribute_code}m
Here, \033[ is the escape sequence, and {attribute_code} is the code that represents the desired text attribute. For example, 31 is the code for red foreground color, and 42 is the code for green background color.
Basic Color Codes
Here are some basic ANSI color codes for foreground and background colors:
Foreground Colors:
Black: 30
Red: 31
Green: 32
Yellow: 33
Blue: 34
Magenta: 35
Cyan: 36
White: 37
Background Colors:
Black: 40
Red: 41
Green: 42
Yellow: 43
Blue: 44
Magenta: 45
Cyan: 46
White: 47
Creating Colored Output in Shell Scripts
To print colored output in a shell script, simply wrap your text with the appropriate ANSI escape codes. For example, to print red text, you can use:
echo -e "\033[31mHello, Red World!\033[0m"
The -e flag in the echo command tells it to interpret backslash escapes.
Reusable Functions for Colored Output
To make it easier to work with colors in shell scripts, you can create reusable functions for different text styles. Here’s an example:
#!/bin/bash
# Color Functions
color_red() {
echo -e "\033[31m${1}\033[0m"
}
color_green() {
echo -e "\033[32m${1}\033[0m"
}
color_yellow() {
echo -e "\033[33m${1}\033[0m"
}
color_blue() {
echo -e "\033[34m${1}\033[0m"
}
# Usage
color_red "This is red text"
color_green "This is green text"
color_yellow "This is yellow text"
color_blue "This is blue text"
Conclusion:
Adding colors to your shell scripts can make them more visually appealing and user-friendly. By utilizing ANSI escape codes, you can customize the text style in your terminal output. With reusable functions, you can easily incorporate colored output into your scripts and create a more engaging user experience.